I have used numpy in python but now I know how these are fast. But, note that there are some important distinctions between them. The concurrency still has some problems: There are two options for creating a Thread in Java. Unit of concurrency: Multiprocessing, Multitasking, Multithreading, Thread in Java: Executor framework and Thread Pool, Thread Synchronization, Locks and Atomic Variables, How to use Parallelism in Java? It is appropriate when you need to return a result from your task, e.g. Lets see a quick example on the application of the Fork/Join Framework. Threads within a process share the processs resources including memory and open files. Part of theParallel, Concurrent, and Distributed Programming in Java Specialization. An increasing number of programming languages (including Java and C++) are moving from older thread-based approaches to more modern task-based approaches for parallel programming. A thread is a path of execution within a process. Parallel, concurrent, and distributed programming underlies software in multiple domains, ranging from biomedical research to financial services. It basically divides a task into smaller subtasks; then, each subtask is further divided into sub-subtasks. In this module, we will learn the fundamentals of task parallelism. Java SE provides the Fork/Join Framework which helps you to more easily implement parallel computing in your applications. This includes coverage of software management systems and project management (PM) software - all aimed at helping to shorten the software development lifecycle (SDL). To create a parallel stream, invoke the operation Collection.parallelStream. It actually involves dividing a problem into subproblems, solving those problems simultaneously, and then combining the results of the solutions to the subproblems. Suppose we are to increment the values of an array of. This type of programming is optimized for a multicore CPU environment. Welcome to Module 3, and congratulations on reaching the midpoint of this course! Developer.com features tutorials, news, and how-tos focused on topics relevant to software engineers, web developers, programmers, and product managers of development teams. .average() All computers are multicore computers, so it is important for you to learn how to extend your knowledge of sequential Java programming to multicore parallelism. Online Master of Data Science | Online Master of Computer Science | Online Master of Engineering Mgmt & Leadership. Welcome to Parallel Programming in Java! The default thread has priority: Thread.NORM_PRIORITY. Give you some case studies, Some notes when we use concurrency and parallelism. In programming, concurrency is the composition of independently executing processes, while parallelism is the simultaneous execution of (possibly related) computations. In a non-parallel environment, what we had to do is cycle through the entire array and do the processing in sequence. We will also learn popular Java APIs for task parallelism, most notably the Fork/Join framework. Visit the Learner Help Center.
However, with this framework, you want to specify how the issues are subdivided (partitioned). The tasks are defined according to the function they perform or data used in processing; this is called functional parallelism or data parallelism, respectively. If there is only one CPU, it is time shared. This, in essence, leads to a tremendous boost in the performance and efficiency of the programs in contrast to linear single-core execution or even multithreading. Parallel programming enables developers to use multicore computers to make their applications run faster by using multiple processors at the same time. Although everything seems hunky-dory with multicore CPUs, here is another side of the story as well. Theory of parallelism: work, span, Amdahls Law, weak vs. strong scaling, data races, determinism, Task parallelism using Javas ForkJoin framework, Functional parallelism using Javas Future interface, Loop-level parallelism using Java 8 Streams, Dataflow parallelism using data-driven tasks. But they are there, unignorablydual, quad, octa, and so on. As far as I know, concurrency has three levels: In this article, we will only discuss the Multithreading level. In short, multiple CPU cores in daily computing provide performance improvement that cannot outweigh the amount of resources needed to use it. Property of TechnologyAdvice. Through a collection of three courses (which may be taken in any order or separately), you will learn foundational topics in Parallelism, Concurrency, and Distribution. It basically divides a task into smaller subtasks; then, each subtask is further divided into sub-subtasks. The instructor, Prof. Vivek Sarkar, would like to thank Dr. Max Grossman for his contributions to the mini-projects and other course material, Dr. Zoran Budimlic for his contributions to the quizzes, Dr. Max Grossman and Dr. Shams Imam for their contributions to the pedagogic PCDP library used in some of the mini-projects, and all members of the Rice Online team who contributed to the development of the course content (including Martin Calvi, Annette Howe, Seth Tyger, and Chong Zhou). Required fields are marked *, The Fork/Join Framework has been defined in the. Dataflow parallelism using the Phaser framework and data-driven tasks The greatest advantage of multithreading is that it is a technique to derive the most out of the processing resources. threading multi java Why take this course? These concepts are very important and complex with every developer. Guess what; it sounds familiar, doesnt it? Why we should know concurrency and parallelism? One difficulty in implementing parallelism in applications that use collections is that collections arent thread-safe, which means that multiple threads cant manipulate a collection without introducing thread interference or memory consistency errors. Your electronic Certificate will be added to your Accomplishments page - from there, you can print your Certificate or add it to your LinkedIn profile. Not that parallelism isnt automatically faster than performing operations serially, although it can be if you have enough data and processor cores. Mastery of these concepts will enable you to immediately apply them in the context of multicore Java programs, and will also help you master other parallel programming systems that you may encounter in the future (e.g., C++11, OpenMP, .Net Task Parallel Library). Suppose we are to increment the values of an array of N numbers. We will learn how Javas Phaser API can be used to implement fuzzy barriers, and also point-to-point synchronizations as an optimization of regular barriers by revisiting the iterative averaging example. What are concurrency and parallelism? This is clearly an inefficient approach in view of parallel processing. Definitely NO! With aggregate operations, the Java runtime performs this partitioning and mixing of solutions for you. It is an implementation of the ExecutorService interface that helps you take advantage of multiple processors. I have never known these things existed and changed my perspective how to take advantage of multiple processor. When a stream is executing in parallel, the Java runtime partitions the stream into multiple substreams. You can use, If you want to run some background tasks asynchronously and wanna return something. Advocates of parallel functional programming have argued for decades that functional parallelism can eliminate many hard-to-detect bugs that can occur with imperative parallelism. For example, the following statement calculates the average age of all male members in parallel: I highly recommend you use them in your project: If you dont wanna return anything from your callback and just wanna run some codes after the completion of CompletableFuture you can use: You can combine two dependent futures using. You can create a Completable simply by using the following no-arg constructor: If you want to run some background tasks asynchronously and dont wanna return anything from the task. For every method call, one entry will be added in the stack called a stack frame. I can see the utilisation of parallel computing in many fields of software development industry.
These properties include work, span, ideal parallelism, parallel speedup, and Amdahls Law. All Rights Reserved Finally, we will also learn how pipeline parallelism and data flow models can be expressed using Java APIs. For an interview with two early-career software engineers on the relevance of parallel computing to their jobs, click here. Task parallelism using Javas ForkJoin framework to initialize a big array with some custom values. This specialization is intended for anyone with a basic knowledge of sequential programming in Java, who is motivated to learn how to write parallel, concurrent and distributed programs. Join Professor Vivek Sarkar as he talks with Two Sigma Managing Director, Jim Ward, and Software Engineers, Margaret Kelley and Jake Kornblau, at their downtown Houston, Texas office about the importance of parallel programming. Java 7 and Java 8 have introduced new frameworks for parallelism (ForkJoin, Stream) that have significantly changed the paradigms for parallel programming since the early days of Java. Yes. In other words, Parallel computing involves dividing a problem into subproblems, solving those problems simultaneously(in parallel, with each subproblem running in a separate thread), and then combining the results of the solutions to the subproblems. There are lots of examples in the real about concurrency. The next two videos will showcase the importance of learning about Concurrent Programming and Distributed Programming in Java.
Its very flexible. We can apply concurrency and parallelism in asynchronous programming. Even the most inexpensive machine is mounted with multicore CPUs. In select learning programs, you can apply for financial aid or a scholarship if you cant afford the enrollment fee. The added advantage with this framework is to use multithreading in a parallel execution environment. To access graded assignments and to earn a Certificate, you will need to purchase the Certificate experience, during or after your audit. .mapToInt(Person::getAge) Welcome to Module 2! The problem of having multiple CPUs is that it requires memory that must match up speed with processing power, along with lightning fast data channels and other accessories. As far as I know about synchronous and asynchronous programming. java Here, the processing part is optimised to use multiple processors unlike multithreading, where the idle time of the single CPU is optimised on the basis of shared time. If you have any doubts/questions, please comment here !!!. This is the task. In this way, we can apply adivide-and-conquerstrategy recursively until the tasks are singled out into a unit problem. CompletableFuture is used for asynchronous programming in Java. Mastery of these concepts will enable you to immediately apply them in the context of multicore Java programs, and will also provide the foundation for mastering other parallel programming systems that you may encounter in the future (e.g., C++11, OpenMP, .Net Task Parallel Library). Unlike multithreading, where each task is a discrete logical unit of a larger task, parallel programming tasks are independent and their execution order does not matter. Back to: Java Tutorials For Beginners and Professionals. Reset deadlines in accordance to your schedule. Rice has highly respected schools of Architecture, Business, Continuing Studies, Engineering, Humanities, Music, Natural Sciences and Social Sciences and is home to the Baker Institute for Public Policy. A thread is only executing one task at a time. When you create a stream, it is always a serial stream unless otherwise specified. Love podcasts or audiobooks? We will start by learning how parallel counted-for loops can be conveniently expressed using forall and stream APIs in Java, and how these APIs can be used to parallelize a simple matrix multiplication program. sort counting parallel figure Parallel programming has a much wider connotation and undoubtedly is a vast area to elaborate in a few lines. This law stipulates that there will always be a maximum speedup that can be achieved when the execution of a program is split into parallel threads. And then, wrap this code in aForkJoinTasksubclass, typically using one of its abstract tasks: It does not return any result; you can use it e.g. double average = roster In this way, we can apply a divide-and-conquer strategy recursively until the tasks are singled out into a unit problem.
Advertiser Disclosure: Some of the products that appear on this site are from companies from which TechnologyAdvice receives compensation. Theory of parallelism: computation graphs, work, span, ideal parallelism, parallel speedup, Amdahl's Law, data races, and determinism These courses will prepare you for multithreaded and distributed programming for a wide range of computer platforms, from mobile devices to cloud computing servers. The main thread can create additional threads within the process. Instructor is awesome. This course teaches learners (industry professionals and students) the fundamental concepts of parallel programming in the context of Java 8. It consists of several classes and interfaces that support parallel programming. The Fork/Join Framework is defined in the java.util.concurrent package. However, each task (includes subtasks) is completed before the next task is split up and executed in parallel.
I hope you enjoy this Parallel Programming in Java with Examplesarticle. They are programming models. Finally, an application can also be both concurrent and parallel. The course may offer 'Full Course, No Certificate' instead. This course is designed as a three-part series and covers a theme or body of knowledge through various video lectures, demonstrations, and coding projects. And then, wrap this code in aForkJoinTasksubclass, typically using one of its abstract tasks: RecursiveActionandRecursiveTask. Each of the four modules in the course includes an assigned mini-project that will provide you with the necessary hands-on experience to use the concepts learned in the course on your own, after the course ends. In the next article, I am going to discussReflection in Javawith Examples. This option lets you see all course materials, submit required assessments, and get a final grade. We also can pause a Thread via sleep() method and waiting for the completion of another thread via the join() method. With the advent of multicore CPUs in recent years, parallel programming is the way to take full advantage of the new processing work horses. The OS keeps track of all these processes and facilitates their execution by sharing the processing time of the CPU among them. We can use the synchronized keyword for method or block. Typically, a task is created with the help of the, It provides a common pool to manage the execution of, This framework uses a divide-and-conquer strategy to implement parallel processing. Happy coding . Parallel Programming Basics with the Fork/Join Framework in Java, ClickUP Project Management Software Review, Agile Frameworks: Scrum vs Kanban vs Lean vs XP, Python Database Programming with MongoDB for Beginners, Python Database Programming with SQL Express for Beginners. As a result, when the tasks are distributed among processors, they can obtain the result relatively fast. Its complements limitations of Future such as: cant be manually completed, cant perform further action on a Futures result without blocking, multiple futures cant be chained together, you cant combine multiple Futures together, no exception handling. If fin aid or scholarship is available for your learning program selection, youll find a link to apply on the description page. The course may not offer an audit option. Parallel programming is suitable for a larger problem base that does not fit into a single CPU architecture, or it may be the problem is so large that it cannot be solved in a reasonable estimate of time.
For example, the following statement calculates the average age of all male members in parallel: .filter(p -> p.getGender() == Person.Sex.MALE), In the next article, I am going to discuss.
Could be less examples with custom libraries instead of standard java features looking to practical usage at work. Acknowledgments If you don't see the audit option: What will I get if I subscribe to this Specialization? This is an abstract class that defines a task. Your email address will not be published. It means that it works on multiple tasks at the same time, and also breaks each task down into subtasks for parallel execution. Any questions?
Within a Java application, you work with several threads to achieve parallel processing or asynchronous behavior. A process is a program in execution. Suppose we are to increment the values of an array of N numbers. We can execute streams in serial or in parallel. During the course, you will have online access to the instructor and the mentors to get individualized answers to your questions posted on forums. Thank you for your reading !!!. In any case, the Java API is not short of its support. Currently, Im developing enterprise web applications that use the Spring Boot project. Learn on the go with our new app. More details: https://docs.oracle.com/javase/8/docs/api/java/util/concurrent/atomic/package-summary.html. This framework enables programmers to parallelize algorithms. Finally, you should use concurrency and parallelism wisely. It also has a very comprehensive exception handling support. Tasks are the most basic unit of parallel programming. Consequently, we get an underutilised expensive machine, perhaps meant only to be showcased. You can check via command line: java -XX:+PrintFlagsFinal -version | grep ThreadStackSize. Java Concurrency API defines three executor interfaces that cover everything that is needed for creating and managing threads: Most of the executor implementations use thread pools to execute tasks. Asynchronous programming is a means of writing non-blocking code by running a task on a separate thread than the main application thread and notifying the main thread about its progress, completion of failure.
It means that the application only works on one task at a time, and this task is broken down into subtasks which can be processed in parallel. (JaiChai) Learning to CodeFocus on the Why instead of the How, Case Study: Spotify migrating to Kubernetes, 5 Questions to Consider before Development of Mobile Apps for IoT, CSS Basics #3: Position, Overflow and Alignment, How to create a Singleton class in Kotlin. Instead of using an intrinsic lock via the synchronized keyword, you can also use various Locking classes provided by Java Concurrency API: ReentrantLock(since java 1.5), ReadWriteLock(since java 1.5), StampedLock(since java 1.8) and Atomic Variables(since java 1.5): AtomicInteger, AtomicBoolean, AtomicLong, AtomicReference and so on. By the end of this course, you will learn how to use popular parallel Java frameworks such as ForkJoin and Stream to write parallel programs for a wide range of multicore platforms whether for servers, desktops, or mobile devices, while also learning about their theoretical foundations (e.g., deadlock freedom, data race freedom, determinism). Is means that it works on only one task at a time, and the task is never broken down into subtasks for parallel execution. Concurrency is about dealing with lots of things at once. In my option, you should use a Runnable object to creating a Thread. It almost rhymes with the idea of multithreading. In fact, multithreading was introduced to provide a sort of illusion of parallel processing with no real parallel execution at all. Parallel programming enables developers to use multicore computers to make their applications run faster by using multiple processors at the same time. This course teaches industry professionals and students the fundamental concepts of parallel programming in the context of Java 8. It is well known that many applications spend a majority of their execution time in loops, so there is a strong motivation to learn how loops can be sped up through the use of parallelism, which is the focus of this module. Parallelism is about doing lots of things at once. Source: blog.golang.org, Concurrency is about structure, parallelism is about execution.. We will also learn about the barrier construct for parallel loops, and illustrate its use with a simple iterative averaging program example. I found this course very useful. Synchronization & Locks: In a multiple-thread program, access to shared variables must be synchronized in order to prevent race conditions.
Access to lectures and assignments depends on your type of enrollment. This, in essence, leads to a tremendous boost in performance and efficiency of the programs in contrast to linear single core execution or even multithreading. This unit problem then can be executed in parallel by the multiple core processors available. In short, multithreading is a collection of discrete logical units of tasks that run to grab their share of CPU time while another thread may be temporarily waiting for, say, some user input. If you mark a variable as volatile the compiler wont optimize or reorder instructions around that variable. Parallel programming code is a difficult frame. Of course, the runtime is limited by parts of the task which can be performed in parallel. TechnologyAdvice does not include all companies or all types of products available in the marketplace. This is clearly an inefficient approach in view of parallel processing.
To create a RecursiveAction, you need to create your own class which extends from. Every process has at least one thread called the main thread.
Unlike multithreading, where each task is a discrete logical unit of a larger task, parallel programming tasks are independent and their execution order does not matter. If there are multiple CPU cores, they also are all time shared. The desired learning outcomes of this course are as follows: An application can also be parallel but not concurrent. Java SE provides the fork/join framework, which enables you to more easily implement parallel programming in your applications. Most of todays machines use multicore CPUs. Additionally, an application can be neither concurrent nor parallel. When will I have access to the lectures and assignments? What it does primarily is that it simplifies the process of multiple thread creation, their uses, and automates the mechanism of process allocation among multiple processors. Aggregate operations iterate over and process these substreams in parallel then combine the results.
scala language programming lang alwaysdata So it's really useful for developers. Only a section of Java applications effectively use this feature. This process is applied recursively to each task until it is small enough to be handled sequentially. Loop-level parallelism with extensions for barriers and iteration grouping (chunking) A computer system normally has multiple processes running at a time.
To call a RecursiveAction, you need to create a new instance of your RecursiveAction implementation and invoke it using ForkJoinPool. In this article, I am going to discussParallel Programming in Java with Examples. More details: https://docs.oracle.com/javase/8/docs/api/java/util/concurrent/CompletableFuture.html. More questions? Parallel programming is suitable for a larger problem base that does not fit in to a single CPU architecture, or it may be the problem is so large that it cannot be solved in a reasonable estimate of time. The crux of the matter is quite simple, yet operationally much harder to achieve. It provides a huge set of convenience methods for creating, chaining, and combining multiple Futures. Advertise with TechnologyAdvice on Developer.com and our other developer-focused platforms.
With the advent of multicore CPUs in recent years, parallel programming is the way to take full advantage of the new processing workhorses. All computers are multicore computers, so it is important for you to learn how to extend your knowledge of sequential Java programming to multicore parallelism. Now, this idea is quite useful and can be used in any set of environments, whether it has a single CPU or multiple CPUs. In addition to covering the most popular programming languages today, we publish reviews and round-ups of developer tools that help devs reduce the time and money spent developing, maintaining, and debugging their applications. Besides, improve significantly the throughput by increasing CPU utilization. sorting a really huge array. But, there are problems that often involve some sort of array, collection, of grouping of data that particularly suits this approach. Box 1892, Houston, TX 77251-1892 |, 713-348-0000 | Privacy Policy | Campus Carry, Online Master of Engineering Mgmt & Leadership, Master of Computer Science Online Program, Master of Engineering Management and Leadership Online Program, Master of Business Administration Online Program, Parallel, Concurrent, and Distributed Programming in Java Specialization. Your email address will not be published. 2022 TechnologyAdvice. This is the task.
Each thread is created in Java 8 will consume about 1MB as default on OS 64 bit.
More details: Parallelism and Fork/Join Framework. There are four core classes in this framework: This framework employs a recursive divide-and-conquer strategy to implement parallel processing. jr programming language concurrent olsson ucdavis cs research edu No harm there. Alternatively, invoke the operation BaseStream.parallel. You can try a Free Trial instead, or apply for Financial Aid. Unlike multithreading, where each task is a discrete logical unit of a larger task, parallel programming tasks are independent and their execution order doesnt matter. 2022 Coursera Inc. All rights reserved. At the end of this article, you will understand what is Parallel Programming and why need Parallel Programming as well as How to implement Parallel Programming in Java with Examples. .filter(p -> p.getGender() == Person.Sex.MALE) Very useful course about parallel programming theory and practice. Therefore, an optimal multithreaded program squeezes out the CPUs performance by the clever mechanism of time sharing. It means that it can process more than one task at the same time, but no two tasks are executing at the exact same time. In simple terms, parallel programming means writing programs that use more than one processor to complete a task, thats all! By the way, there are problems that may not use collection of data yet can be optimised to use the strategy for parallel programming. In reality, it is relatively difficult to keep even a single CPU busy in daily computing. https://blog.golang.org/waza-talk#:~:text=In%20programming%2C%20concurrency%20is%20the,lots%20of%20things%20at%20once.
However, with this framework, you want to specify how the issues are subdivided (partitioned). The tasks are defined according to the function they perform or data used in processing; this is called functional parallelism or data parallelism, respectively. If there is only one CPU, it is time shared. This, in essence, leads to a tremendous boost in the performance and efficiency of the programs in contrast to linear single-core execution or even multithreading. Parallel programming enables developers to use multicore computers to make their applications run faster by using multiple processors at the same time. Although everything seems hunky-dory with multicore CPUs, here is another side of the story as well. Theory of parallelism: work, span, Amdahls Law, weak vs. strong scaling, data races, determinism, Task parallelism using Javas ForkJoin framework, Functional parallelism using Javas Future interface, Loop-level parallelism using Java 8 Streams, Dataflow parallelism using data-driven tasks. But they are there, unignorablydual, quad, octa, and so on. As far as I know, concurrency has three levels: In this article, we will only discuss the Multithreading level. In short, multiple CPU cores in daily computing provide performance improvement that cannot outweigh the amount of resources needed to use it. Property of TechnologyAdvice. Through a collection of three courses (which may be taken in any order or separately), you will learn foundational topics in Parallelism, Concurrency, and Distribution. It basically divides a task into smaller subtasks; then, each subtask is further divided into sub-subtasks. The instructor, Prof. Vivek Sarkar, would like to thank Dr. Max Grossman for his contributions to the mini-projects and other course material, Dr. Zoran Budimlic for his contributions to the quizzes, Dr. Max Grossman and Dr. Shams Imam for their contributions to the pedagogic PCDP library used in some of the mini-projects, and all members of the Rice Online team who contributed to the development of the course content (including Martin Calvi, Annette Howe, Seth Tyger, and Chong Zhou). Required fields are marked *, The Fork/Join Framework has been defined in the. Dataflow parallelism using the Phaser framework and data-driven tasks The greatest advantage of multithreading is that it is a technique to derive the most out of the processing resources. threading multi java Why take this course? These concepts are very important and complex with every developer. Guess what; it sounds familiar, doesnt it? Why we should know concurrency and parallelism? One difficulty in implementing parallelism in applications that use collections is that collections arent thread-safe, which means that multiple threads cant manipulate a collection without introducing thread interference or memory consistency errors. Your electronic Certificate will be added to your Accomplishments page - from there, you can print your Certificate or add it to your LinkedIn profile. Not that parallelism isnt automatically faster than performing operations serially, although it can be if you have enough data and processor cores. Mastery of these concepts will enable you to immediately apply them in the context of multicore Java programs, and will also help you master other parallel programming systems that you may encounter in the future (e.g., C++11, OpenMP, .Net Task Parallel Library). Suppose we are to increment the values of an array of N numbers. We will learn how Javas Phaser API can be used to implement fuzzy barriers, and also point-to-point synchronizations as an optimization of regular barriers by revisiting the iterative averaging example. What are concurrency and parallelism? This is clearly an inefficient approach in view of parallel processing. Definitely NO! With aggregate operations, the Java runtime performs this partitioning and mixing of solutions for you. It is an implementation of the ExecutorService interface that helps you take advantage of multiple processors. I have never known these things existed and changed my perspective how to take advantage of multiple processor. When a stream is executing in parallel, the Java runtime partitions the stream into multiple substreams. You can use, If you want to run some background tasks asynchronously and wanna return something. Advocates of parallel functional programming have argued for decades that functional parallelism can eliminate many hard-to-detect bugs that can occur with imperative parallelism. For example, the following statement calculates the average age of all male members in parallel: I highly recommend you use them in your project: If you dont wanna return anything from your callback and just wanna run some codes after the completion of CompletableFuture you can use: You can combine two dependent futures using. You can create a Completable simply by using the following no-arg constructor: If you want to run some background tasks asynchronously and dont wanna return anything from the task. For every method call, one entry will be added in the stack called a stack frame. I can see the utilisation of parallel computing in many fields of software development industry.
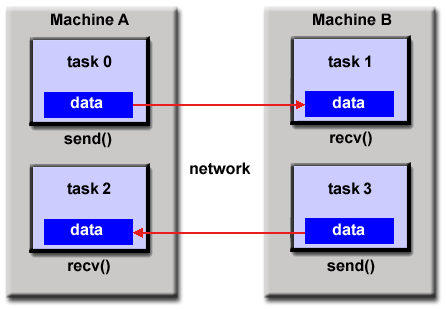

Advertiser Disclosure: Some of the products that appear on this site are from companies from which TechnologyAdvice receives compensation. Theory of parallelism: computation graphs, work, span, ideal parallelism, parallel speedup, Amdahl's Law, data races, and determinism These courses will prepare you for multithreaded and distributed programming for a wide range of computer platforms, from mobile devices to cloud computing servers. The main thread can create additional threads within the process. Instructor is awesome. This course teaches learners (industry professionals and students) the fundamental concepts of parallel programming in the context of Java 8. It consists of several classes and interfaces that support parallel programming. The Fork/Join Framework is defined in the java.util.concurrent package. However, each task (includes subtasks) is completed before the next task is split up and executed in parallel.
For example, the following statement calculates the average age of all male members in parallel: .filter(p -> p.getGender() == Person.Sex.MALE), In the next article, I am going to discuss.
Could be less examples with custom libraries instead of standard java features looking to practical usage at work. Acknowledgments If you don't see the audit option: What will I get if I subscribe to this Specialization? This is an abstract class that defines a task. Your email address will not be published. It means that it works on multiple tasks at the same time, and also breaks each task down into subtasks for parallel execution. Any questions?
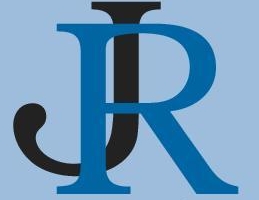
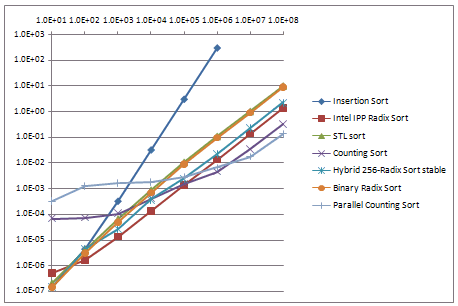
Access to lectures and assignments depends on your type of enrollment. This, in essence, leads to a tremendous boost in performance and efficiency of the programs in contrast to linear single core execution or even multithreading. This unit problem then can be executed in parallel by the multiple core processors available. In short, multithreading is a collection of discrete logical units of tasks that run to grab their share of CPU time while another thread may be temporarily waiting for, say, some user input. If you mark a variable as volatile the compiler wont optimize or reorder instructions around that variable. Parallel programming code is a difficult frame. Of course, the runtime is limited by parts of the task which can be performed in parallel. TechnologyAdvice does not include all companies or all types of products available in the marketplace. This is clearly an inefficient approach in view of parallel processing.
To create a RecursiveAction, you need to create your own class which extends from. Every process has at least one thread called the main thread.
Unlike multithreading, where each task is a discrete logical unit of a larger task, parallel programming tasks are independent and their execution order does not matter. If there are multiple CPU cores, they also are all time shared. The desired learning outcomes of this course are as follows: An application can also be parallel but not concurrent. Java SE provides the fork/join framework, which enables you to more easily implement parallel programming in your applications. Most of todays machines use multicore CPUs. Additionally, an application can be neither concurrent nor parallel. When will I have access to the lectures and assignments? What it does primarily is that it simplifies the process of multiple thread creation, their uses, and automates the mechanism of process allocation among multiple processors. Aggregate operations iterate over and process these substreams in parallel then combine the results.
scala language programming lang alwaysdata So it's really useful for developers. Only a section of Java applications effectively use this feature. This process is applied recursively to each task until it is small enough to be handled sequentially. Loop-level parallelism with extensions for barriers and iteration grouping (chunking) A computer system normally has multiple processes running at a time.
To call a RecursiveAction, you need to create a new instance of your RecursiveAction implementation and invoke it using ForkJoinPool. In this article, I am going to discussParallel Programming in Java with Examples. More details: https://docs.oracle.com/javase/8/docs/api/java/util/concurrent/CompletableFuture.html. More questions? Parallel programming is suitable for a larger problem base that does not fit in to a single CPU architecture, or it may be the problem is so large that it cannot be solved in a reasonable estimate of time. The crux of the matter is quite simple, yet operationally much harder to achieve. It provides a huge set of convenience methods for creating, chaining, and combining multiple Futures. Advertise with TechnologyAdvice on Developer.com and our other developer-focused platforms.
With the advent of multicore CPUs in recent years, parallel programming is the way to take full advantage of the new processing workhorses. All computers are multicore computers, so it is important for you to learn how to extend your knowledge of sequential Java programming to multicore parallelism. Now, this idea is quite useful and can be used in any set of environments, whether it has a single CPU or multiple CPUs. In addition to covering the most popular programming languages today, we publish reviews and round-ups of developer tools that help devs reduce the time and money spent developing, maintaining, and debugging their applications. Besides, improve significantly the throughput by increasing CPU utilization. sorting a really huge array. But, there are problems that often involve some sort of array, collection, of grouping of data that particularly suits this approach. Box 1892, Houston, TX 77251-1892 |, 713-348-0000 | Privacy Policy | Campus Carry, Online Master of Engineering Mgmt & Leadership, Master of Computer Science Online Program, Master of Engineering Management and Leadership Online Program, Master of Business Administration Online Program, Parallel, Concurrent, and Distributed Programming in Java Specialization. Your email address will not be published. 2022 TechnologyAdvice. This is the task.
More details: Parallelism and Fork/Join Framework. There are four core classes in this framework: This framework employs a recursive divide-and-conquer strategy to implement parallel processing. jr programming language concurrent olsson ucdavis cs research edu No harm there. Alternatively, invoke the operation BaseStream.parallel. You can try a Free Trial instead, or apply for Financial Aid. Unlike multithreading, where each task is a discrete logical unit of a larger task, parallel programming tasks are independent and their execution order doesnt matter. 2022 Coursera Inc. All rights reserved. At the end of this article, you will understand what is Parallel Programming and why need Parallel Programming as well as How to implement Parallel Programming in Java with Examples. .filter(p -> p.getGender() == Person.Sex.MALE) Very useful course about parallel programming theory and practice. Therefore, an optimal multithreaded program squeezes out the CPUs performance by the clever mechanism of time sharing. It means that it can process more than one task at the same time, but no two tasks are executing at the exact same time. In simple terms, parallel programming means writing programs that use more than one processor to complete a task, thats all! By the way, there are problems that may not use collection of data yet can be optimised to use the strategy for parallel programming. In reality, it is relatively difficult to keep even a single CPU busy in daily computing. https://blog.golang.org/waza-talk#:~:text=In%20programming%2C%20concurrency%20is%20the,lots%20of%20things%20at%20once.