MySQL is an open-source relational database management system. Shopify Hydrogen: Why is useShopQuery server-side only? The validate request middleware function validates the body of a request against a Joi schema object. Hope, it helped in adding value to your knowledge. Made with love and Ruby on Rails. A techno freak who likes to explore Sr Research Analyst at Edureka. Then navigate to NodeMysqlCrudApp directory. Finally, lets create the DELETE router. Further to this if a custom error ends with the words 'not found' a 404 response code is returned, otherwise a standard 400 response is returned. Or maybe complement the post with that? If validation succeeds the request continues to the next middleware function (the route function), otherwise an error is returned with details of why validation failed. The users folder contains all code that is specific to the users feature of the Node.js + MySQL CRUD API. Next, you need to create a router to update the learner details.
The MySQL database wrapper connects to MySQL using Sequelize & the MySQL2 client, and exports an object that exposes all of the database models for the application (currently only User). res.json({error:false,message:"Employee added successfully! Run our Node.js application with command: node server.js. After that, use the connect() function to connect establish the connection with the database using provided credentials. What do you think about it? Index.ejs file, we will display the list of customers. Its name is a combination of My, the name of co-founder Michael Wideniuss daughter, and SQL, the abbreviation for Structured Query Language. It is used by schema middleware functions in controllers to validate requests with a schema for a specific route (e.g.
Next follow the below commands and install node js in your project : Install flash,validator,session ,override MySQL Libraries into your node js express crud + MySQL application by executing the following command on terminal: Flash is an extension of connect-flash with the ability to define a flash message and render it without redirecting the request.In this node js mysql crud tutorial express flash is used to display a warning, error and information message. Just to crosscheck, you can open your workbench and hit the refresh button to see the new record in the table. Subscribe to Feed: But to get up and running quickly just follow the below steps. Do you plan to write about "best practice in REST API" or "Soft delete" With Node.js? For further actions, you may consider blocking this person and/or reporting abuse. You will recieve an email from us shortly. Now open db.config.js and add code below for creating mysql connection. Since my main aim is to demonstrate data transactions from the database, I will be mainly focussing on the controllers. laravel inserted bootstrap minus The, A code editor to view and edit the API code, it doesn't matter which one, personally I use, Download or clone the project source code from, Install all required npm packages by running, You can test the API directly with a tool such as, Download or clone the React tutorial code from, Remove or comment out the 2 lines below the comment, Download or clone the Angular tutorial code from, Remove or comment out the line below the comment. Refer the below code: I will be using another application to make my requests called Postman. Now when you try to fetch the complete list of the learners, you will see the newly inserted record as well.
I share tutorials of PHP, Python, Javascript, JQuery, Laravel, Livewire, Codeigniter, Node JS, Express JS, Vue JS, Angular JS, React Js, MySQL, MongoDB, REST APIs, Windows, Xampp, Linux, Ubuntu, Amazon AWS, Composer, SEO, WordPress, SSL and Bootstrap from a starting stage. To know more about it, you can refer to this article on, Here I will be creating a simple CRUD application using Node.js and link it with, As you can see, in the dependencies section all the installed packages have been successfully listed. Now its time to create our project. Postman is an API(application programming interface) development tool which helps to build, test and modify APIs.It has the ability to make various types of HTTP requests(GET, POST, PUT, PATCH etc.). Type in the below codes to define all the required routines: Once done, switch back to the script.js file, and type in the below code for the POST request. The CRUD API project is available on GitHub at https://github.com/cornflourblue/node-mysql-crud-api. After successfully created expressfirst folder in your system. To make connectivity with database in our project well make seperate file. In very simple terms, MySQL is an open-source relational database management system which can operate on various platforms. const Employee = require('../models/employee.model'); Employee.findAll(function(err, employee) {. If you want to gain more insights on Node.js you can refer to my, Join Edureka Meetup community for 100+ Free Webinars each month. In which you can add customers, edit customers and also delete customers from the database using node js express with MySQL database. Finally package.json looks like below. Now you need to set up the project configurations for that, type in the below command and provide the necessary details: Now, you need to install the required packages. ", [id], function (err, res) {. Below is the detailed list of the topics that will be discussed in this Node.js MySQL Tutorial: Let me start off this Node.js MySQL tutorial by addressing the most basic question i.e why do we use MySQL. First, we start with an Express web server. For that open up MySQL workbench. Built on Forem the open source software that powers DEV and other inclusive communities.
Node.js NPM Tutorial How to Get Started with NPM? The user model uses Sequelize to define the schema for the users table in the MySQL database. Here you need to specify what Node.js should do if the connection is successful and what should be done if the connection fails. By convention errors of type 'string' are treated as custom (app specific) errors, this simplifies the code for throwing custom errors since only a string needs to be thrown (e.g. An IDE normally consists of at least a source code editor, build automation tools, and a debugger. So create a config folder at root and make a db.config.js file inside config folder. The package.json file contains project configuration information including Node.js package dependencies that get installed when you run npm install. employee.status : 1; Employee.create = function (newEmp, result) {, dbConn.query("INSERT INTO employees set ? Hi! If you found this Node.js MySQL Tutorial relevant,check out theNode.js Course by Edureka,a trusted online learning companywith a network of more than250,000satisfied learnersspread acrossthe globe. DEV Community 2016 - 2022. Now if you go back to your MySQL workbench and do a refresh, you will see the particular record has been updated. Templates let you quickly answer FAQs or store snippets for re-use. Lets do some change in package.json file , add a line of code in scripts object of package.json file. Once unpublished, this post will become invisible to the public Your email address will not be published. Next, lets try to create a router to fetch the details of a specific learner by passing in the learners ID. throw 'Error message'). This tutorial will guide you through the steps of building a simple Node.js CRUD Operation with MySQL database using Expressjs for Rest API. IDE (integrated development environment) is a software application that provides comprehensive facilities to computer programmers for software development. At least 1 upper-case and 1 lower-case letter, Minimum 8 characters and Maximum 50 characters. Click any of the below links to jump down to a description of each file along with its code: The helpers folder contains all the bits and pieces that don't fit into other folders but don't justify having a folder of their own. See the user service for some examples of custom errors thrown by the API, errors are caught in the users controller for each route and passed to the next function which passes them to this global error handler. If you want to gain more insights on Node.js you can refer to my other articles on Node.js as well. Hope, it helped in adding value to your knowledge. All rights reserved. For that, you have to use the createConnection() function and provide the required details such as host, user, password and database. res.json({ error:false, message: 'Employee successfully deleted' }); const employeeController = require('../controllers/employee.controller'); router.get('/', employeeController.findAll); router.post('/', employeeController.create); router.get('/:id', employeeController.findById); router.put('/:id', employeeController.update); router.delete('/:id', employeeController.delete); const bodyParser = require('body-parser'); app.use(bodyParser.urlencoded({ extended: true })), const employeeRoutes = require('./src/routes/employee.routes'), app.use('/api/v1/employees', employeeRoutes). console.log(`Server is listening on port ${port}`); https://github.com/rahulguptafullstack/node-mysql-crud-app, GET /api/v1/employees: will give all employees stored in database. The easiest way to install MySQL is by using the MySQL Installer. Now, select GET from the drop-down list and type in the below URL:http://localhost:8080/learners. NPM is used to run a DELETE and PUT method from an HTML form. Under your learner database, you will find a Stored Procedure. Along with this, it is also responsible for invoking the server and establish the connection. The middleware folder contains Express.js middleware functions that can be used by routes / features within the Node.js CRUD API. ",data:employee}); Employee.findById(req.params.id, function(err, employee) {, Employee.update(req.params.id, new Employee(req.body), function(err, employee) {. Next, we add configuration for MySQL database, create Customer model, write the controller. Here I will be creating a simple CRUD application using Node.js and link it with MySQL database to store the data of learners in it.
The scripts section contains scripts that are executed by running the command npm run
The MySQL database wrapper connects to MySQL using Sequelize & the MySQL2 client, and exports an object that exposes all of the database models for the application (currently only User). res.json({error:false,message:"Employee added successfully! Run our Node.js application with command: node server.js. After that, use the connect() function to connect establish the connection with the database using provided credentials. What do you think about it? Index.ejs file, we will display the list of customers. Its name is a combination of My, the name of co-founder Michael Wideniuss daughter, and SQL, the abbreviation for Structured Query Language. It is used by schema middleware functions in controllers to validate requests with a schema for a specific route (e.g.
Next follow the below commands and install node js in your project : Install flash,validator,session ,override MySQL Libraries into your node js express crud + MySQL application by executing the following command on terminal: Flash is an extension of connect-flash with the ability to define a flash message and render it without redirecting the request.In this node js mysql crud tutorial express flash is used to display a warning, error and information message. Just to crosscheck, you can open your workbench and hit the refresh button to see the new record in the table. Subscribe to Feed: But to get up and running quickly just follow the below steps. Do you plan to write about "best practice in REST API" or "Soft delete" With Node.js? For further actions, you may consider blocking this person and/or reporting abuse. You will recieve an email from us shortly. Now open db.config.js and add code below for creating mysql connection. Since my main aim is to demonstrate data transactions from the database, I will be mainly focussing on the controllers. laravel inserted bootstrap minus The, A code editor to view and edit the API code, it doesn't matter which one, personally I use, Download or clone the project source code from, Install all required npm packages by running, You can test the API directly with a tool such as, Download or clone the React tutorial code from, Remove or comment out the 2 lines below the comment, Download or clone the Angular tutorial code from, Remove or comment out the line below the comment. Refer the below code: I will be using another application to make my requests called Postman. Now when you try to fetch the complete list of the learners, you will see the newly inserted record as well.
I share tutorials of PHP, Python, Javascript, JQuery, Laravel, Livewire, Codeigniter, Node JS, Express JS, Vue JS, Angular JS, React Js, MySQL, MongoDB, REST APIs, Windows, Xampp, Linux, Ubuntu, Amazon AWS, Composer, SEO, WordPress, SSL and Bootstrap from a starting stage. To know more about it, you can refer to this article on, Here I will be creating a simple CRUD application using Node.js and link it with, As you can see, in the dependencies section all the installed packages have been successfully listed. Now its time to create our project. Postman is an API(application programming interface) development tool which helps to build, test and modify APIs.It has the ability to make various types of HTTP requests(GET, POST, PUT, PATCH etc.). Type in the below codes to define all the required routines: Once done, switch back to the script.js file, and type in the below code for the POST request. The CRUD API project is available on GitHub at https://github.com/cornflourblue/node-mysql-crud-api. After successfully created expressfirst folder in your system. To make connectivity with database in our project well make seperate file. In very simple terms, MySQL is an open-source relational database management system which can operate on various platforms. const Employee = require('../models/employee.model'); Employee.findAll(function(err, employee) {. If you want to gain more insights on Node.js you can refer to my, Join Edureka Meetup community for 100+ Free Webinars each month. In which you can add customers, edit customers and also delete customers from the database using node js express with MySQL database. Finally package.json looks like below. Now you need to set up the project configurations for that, type in the below command and provide the necessary details: Now, you need to install the required packages. ", [id], function (err, res) {. Below is the detailed list of the topics that will be discussed in this Node.js MySQL Tutorial: Let me start off this Node.js MySQL tutorial by addressing the most basic question i.e why do we use MySQL. First, we start with an Express web server. For that open up MySQL workbench. Built on Forem the open source software that powers DEV and other inclusive communities.
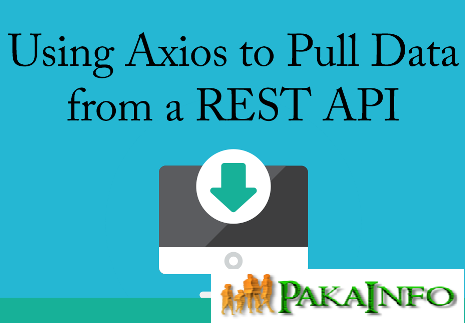
The scripts section contains scripts that are executed by running the command npm run