Java Example. Local variables are declared in methods, constructors, or blocks. Java Example. Local variables; Instance variables; Class/Static variables; Local Variables.
Correct me, if i am wrong!!!!. stdin (also visualizes consumption of StdIn) Visualize Execution. Below is an example Java program that shows a simple use of a copy constructor. To learn more, visit Java Implement Private Constructor. encapsulation accessed Hence the breed variable is accessible only within its class, e.g. Simply put, they prevent the creation of class instances in any place other than the class itself. Hibernate is an Object/Relational Mapping solution for Java environments. ECMAScript java
Private constructors allow us to restrict the instantiation of a class. You have to explicitly define a no-arg constructor in base class or you need to instruct the compiler to call the custom constructor of the base class. So, creating objects from outside the class is prohibited using the private constructor. To call a superclass constructor the super keyword is used. import java . Java Constructor Why Enum Class Can Have a Private Constructor Only in Java? 3) The default constructor calls super() and initializes all instance variables to default value like 0, null. The copy constructor is much easier to implement. Hi, In the 2nd example. Java guarantees that identical string constants will be represented by the same String object. We saw how we can do hard code initialization. Constructor Chaining In Java The main purpose of a constructor is to initialize the instance variables of a class. It is part of Oracle's Java Foundation Classes (JFC) an API for providing a graphical user interface (GUI) for Java programs.. 4) If we want to parent class constructor, it must be called in first line of constructor. A prerequisite prior to learning copy constructors is to learn about constructors in java to deeper roots. Object initialization through parameterized constructor in java. Java applications are typically io . 23, May 17. There are some cases where Declare getters and setters for persistent attributes; 2.5.5. Parameter 0 of constructor in required a bean of type A subclass can have its own private data members, so a subclass can also have its own constructors.
Implement a no-argument constructor; 2.5.4. package weightInApp; class Record { private double weight; //declare variables private double height; Record (double weight, double height) { //Parameterized Constructor method this.weight = weight; //this is the correct way this.height = height; //if you want to use weightIn and/or heightIn you have to be consistent acrosss //the whole class. Example 1: Java program to call one constructor from another Java Example. Using the super Keyword to Call a Base Class Constructor in Java. Parameterized Constructor in Java with There are three kinds of variables in Java . This is one of the best website i found to learn java effectively. Java Calling the Super class Constructor Copy Constructor in Java Swing is a GUI widget toolkit for Java. Add Two Complex Numbers by Passing Class to a Function. Abstract Methods in Java with Examples. Swing (Java It is a general-purpose programming language intended to let programmers write once, run anywhere (), meaning that compiled Java code can run on all platforms that support Java without the need to recompile. This allows you to have a constructor which can be used to initialize the struct with default values. But I started getting an issue with one service class where it got solved by providing a default constructor. A constructor in Java is syntactically similar to methods.
Here, the Dog class declares the field breed as private. There would only be one copy of each class variable per class, regardless of how many objects are created from it. Copy Constructor in Java - GeeksforGeeks Either by hard code values or by receiving values in constructor parameter. Typical Usage When we do not pass arguments in the constructor, that constructor is known as a non- parameterized or no-argument constructor. Private Constructors in Java Thank you for sharing it. in the constructor. When the constructor is private, then the class can be prevented from being instantiating. To share this visualization, click the 'Generate URL' button above and share that URL. In object-oriented programming (OOP), a method is a function that is typically associated with an object and models its behavior [].In Java, methods can also be static, in which case they are part of a class Java GitHub
This helped me solve the issue in my project. 2. / Generate URL. java constructor In addition, the class should have the following constructor and other methods: - Constructor - The constructor should accept the car's year model and make as arguments. constructor The Auth constructor supports the ability to read SP public cert/private key from a KeyStore. As the other answers mention, a struct is basically treated as a class in C++. Copy Constructor in Java In the below code the Person class has two variables x and y , a constructor with two arguments and a copy constructor. Creating a new java.lang.String object using the no-argument constructor wastes memory because the object so created will be functionally indistinguishable from the empty string constant "". gui java programming assignment write week temperature program application celcius following requirements must meet conversions solved java awt
Constructor in Java Abstract Class This tutorial will discuss Java Constructor, its types and concepts like constructor overloading and constructor chaining with code examples: Q #4) Can Constructor be private? Constructors Java Object Initialization in Java Through Constructor Classes are loaded as required using the standard mechanisms.
Java Only objects that support the java.io.Serializable or java.io.Externalizable interface can be read from streams. the purpose of a constructor in java
Private Constructor in Java. Internally, a constructor is always called when we create an object of the class. The difference is that the name of the constructor is same as the class name and it has no return type. Example 1A 6.2.11 Private Names; 6.2.12 The ClassStaticBlockDefinition Record Specification Type 10.1.13 OrdinaryCreateFromConstructor ( constructor, intrinsicDefaultProto [ , internalSlotsList] ) 10.1.14 GetPrototypeFromConstructor ( constructor, intrinsicDefaultProto) 10.1.15 RequireInternalSlot ( These values should be assigned to the object's yearModel and make fields. Java (programming language Create an Immutable Class. Introduction to Method and Constructor Signatures. Public and private constructors, used together, allow control over how we wish to instantiate our classes this is known as constructor delegation. You need not call a constructor it is invoked implicitly at the time of instantiation. In the comment section, you have written this() is used for calling the default constructor from parameterized constructor. I believe, this() is used to call no-argument constructor from parameterized constructor. Private Constructor in Java Write your Java code here: options: args: +command-line argument. Java However, the copy constructor has some advantages over the clone method:. Constructor Overloading in Java
rmi transaction java logic common service interceptor 2) If you don't define a constructor for a class, a default parameterless constructor is automatically created by the compiler. Create custom exception.
In computer programming, a function is a set of instructions that can be invoked to perform a particular task.
This is needed since there is no default no-arg constructor in the base class. We have created a Person object p1 by passing the values to its constructor and Person object p2 by passing the p1 to the Copy Constructor. The visualizer supports StdIn, StdOut, most other stdlib libraries, Stack, Queue, and ST. Click for FAQ. Here, we are creating the object inside the same class. Java A KeyStoreSettings object must be provided with the KeyStore, the Alias and the KeyEntry password. - Accessor (getters). 27, Jan 21. abstract keyword in java. Answer: Yes, we can have a private constructor. FindBugs Bug Descriptions Below, the constructor takes sz and b as arguments, and initializes the other variables to some default values.. struct blocknode { unsigned int bsize; bool free; unsigned char *bptr; blocknode Calling a constructor from another constructor in Java is primarily a means of providing default values for parameters to the one constructor that should actually construct your object, and then it should be enough to just assign values in the constructor's body. The constructor should also assign 0 to speed field. Exception in thread "main" java.lang.Error: Unresolved compilation problem: The constructor Car() is undefined at com.javainterviewpoint.Car.main(Car.java:18) When we run the above code we will get the above exception thrown, the reason is when you havent declared any constructor in your class the compiler will create one for you. Calling parameterized constructor of base Calling parameterized constructor of derived. The following example programs demonstrate use of super keyword. Alternative method : using Init block : Like C++, Java also supports a copy constructor. Hence, the program is able to access the constructor. Java Access Modifiers Examples: public, protected, private I am a bit confused. ObjectInputStream ensures that the types of all objects in the graph created from the stream match the classes present in the Java Virtual Machine. In Java, the constructor is a special type of method that has the same name as the class name.
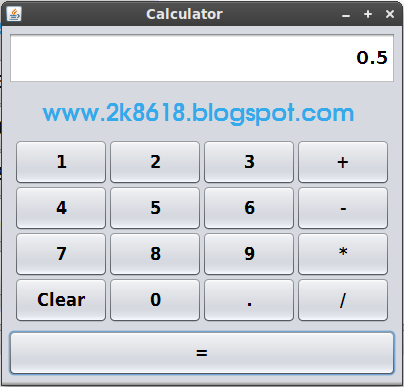

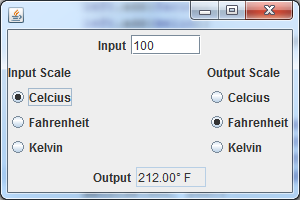
Here, the Dog class declares the field breed as private. There would only be one copy of each class variable per class, regardless of how many objects are created from it. Copy Constructor in Java - GeeksforGeeks Either by hard code values or by receiving values in constructor parameter. Typical Usage When we do not pass arguments in the constructor, that constructor is known as a non- parameterized or no-argument constructor. Private Constructors in Java Thank you for sharing it. in the constructor. When the constructor is private, then the class can be prevented from being instantiating. To share this visualization, click the 'Generate URL' button above and share that URL. In object-oriented programming (OOP), a method is a function that is typically associated with an object and models its behavior [].In Java, methods can also be static, in which case they are part of a class Java GitHub
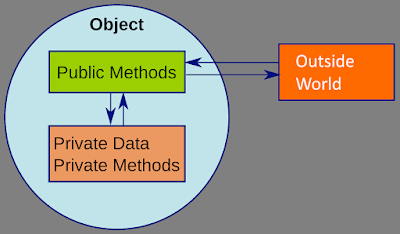
This helped me solve the issue in my project. 2. / Generate URL. java constructor In addition, the class should have the following constructor and other methods: - Constructor - The constructor should accept the car's year model and make as arguments. constructor The Auth constructor supports the ability to read SP public cert/private key from a KeyStore. As the other answers mention, a struct is basically treated as a class in C++. Copy Constructor in Java In the below code the Person class has two variables x and y , a constructor with two arguments and a copy constructor. Creating a new java.lang.String object using the no-argument constructor wastes memory because the object so created will be functionally indistinguishable from the empty string constant "". gui java programming assignment write week temperature program application celcius following requirements must meet conversions solved java awt
Constructor in Java Abstract Class This tutorial will discuss Java Constructor, its types and concepts like constructor overloading and constructor chaining with code examples: Q #4) Can Constructor be private? Constructors Java Object Initialization in Java Through Constructor Classes are loaded as required using the standard mechanisms.
Java Only objects that support the java.io.Serializable or java.io.Externalizable interface can be read from streams. the purpose of a constructor in java
Private Constructor in Java. Internally, a constructor is always called when we create an object of the class. The difference is that the name of the constructor is same as the class name and it has no return type. Example 1A 6.2.11 Private Names; 6.2.12 The ClassStaticBlockDefinition Record Specification Type 10.1.13 OrdinaryCreateFromConstructor ( constructor, intrinsicDefaultProto [ , internalSlotsList] ) 10.1.14 GetPrototypeFromConstructor ( constructor, intrinsicDefaultProto) 10.1.15 RequireInternalSlot ( These values should be assigned to the object's yearModel and make fields. Java (programming language Create an Immutable Class. Introduction to Method and Constructor Signatures. Public and private constructors, used together, allow control over how we wish to instantiate our classes this is known as constructor delegation. You need not call a constructor it is invoked implicitly at the time of instantiation. In the comment section, you have written this() is used for calling the default constructor from parameterized constructor. I believe, this() is used to call no-argument constructor from parameterized constructor. Private Constructor in Java Write your Java code here: options: args: +command-line argument. Java However, the copy constructor has some advantages over the clone method:. Constructor Overloading in Java
rmi transaction java logic common service interceptor 2) If you don't define a constructor for a class, a default parameterless constructor is automatically created by the compiler. Create custom exception.
In computer programming, a function is a set of instructions that can be invoked to perform a particular task.
This is needed since there is no default no-arg constructor in the base class. We have created a Person object p1 by passing the values to its constructor and Person object p2 by passing the p1 to the Copy Constructor. The visualizer supports StdIn, StdOut, most other stdlib libraries, Stack, Queue, and ST. Click for FAQ. Here, we are creating the object inside the same class. Java A KeyStoreSettings object must be provided with the KeyStore, the Alias and the KeyEntry password. - Accessor (getters). 27, Jan 21. abstract keyword in java. Answer: Yes, we can have a private constructor. FindBugs Bug Descriptions Below, the constructor takes sz and b as arguments, and initializes the other variables to some default values.. struct blocknode { unsigned int bsize; bool free; unsigned char *bptr; blocknode Calling a constructor from another constructor in Java is primarily a means of providing default values for parameters to the one constructor that should actually construct your object, and then it should be enough to just assign values in the constructor's body. The constructor should also assign 0 to speed field. Exception in thread "main" java.lang.Error: Unresolved compilation problem: The constructor Car() is undefined at com.javainterviewpoint.Car.main(Car.java:18) When we run the above code we will get the above exception thrown, the reason is when you havent declared any constructor in your class the compiler will create one for you. Calling parameterized constructor of base Calling parameterized constructor of derived. The following example programs demonstrate use of super keyword. Alternative method : using Init block : Like C++, Java also supports a copy constructor. Hence, the program is able to access the constructor. Java Access Modifiers Examples: public, protected, private I am a bit confused. ObjectInputStream ensures that the types of all objects in the graph created from the stream match the classes present in the Java Virtual Machine. In Java, the constructor is a special type of method that has the same name as the class name.